Table of Contents
In the world of web development, having a visually appealing and user-friendly interface is essential. The progress bar is a vital aspect of reaching this goal. Progress bars not only give users a sense of readiness and feedback, but they also enhance the overall user experience. Although there are various ways to implement progress bars, CSS offers a flexible and adaptable approach. In this post, we’ll look into CSS progress bars, including its capabilities, stylistic options, and recommended implementation methods.
Structure of a Circular CSS Progress Bar
At its core, a progress bar is a graphic representation of the completion status of a task or process. CSS allows developers to create progress bars using simple markup and stylistic techniques without relying on complicated JavaScript tools or frameworks. By employing CSS variables such as width, background-color, and border-radius, developers can adjust the appearance of progress bars to correspond with their design preferences and branding requirements.
How a circular Progress Bar Works
A circular progress bar is a visual indicator used in user interfaces to display the status of an operation or process. Circular progress bars fill clockwise around a circle rather than horizontally from left to right.
For what purposes could you employ a Progress Bar?
A CSS progress bar is a graphical representation typically used in software interfaces to display the development of a job or process. Here are some circumstances where a progress bar might be used:
- File Downloads: When downloading a file from the internet, a progress bar can show how much of the file has been downloaded and how much remains.
- File Uploads: Similarly, when uploading a file to a website or server, a progress bar might indicate the upload status.
- Software Installation: A progress bar displays the installation status and remaining time.
- File Copying/Moving: A progress bar can indicate the status of file transfers on a computer.
- Media Playback: In media players, a progress bar indicates how much of a video or audio file has been played and how much is remained.
- Form Submission: In web forms that involve long operations, such as submitting data or uploading files, a progress bar might show the progress of the submission.
- Loading Screens: Progress bars are commonly used to indicate data loading and provide users with an estimated wait time.
- Software Updates: A progress bar indicates the download and installation of updates.
- Data Processing: In applications where huge volumes of data are being processed, such as in data analysis or rendering software, a progress bar might show the progress of the processing activity.
- Multi-step Processes: In multi-step processes, such as wizards or setup tutorials, a progress bar can show users where they are in the process and how many steps left.
4 Amazing CSS Progress Bar Examples
1. CSS Progress Bar styling to create a rotating circle border effect
Output:
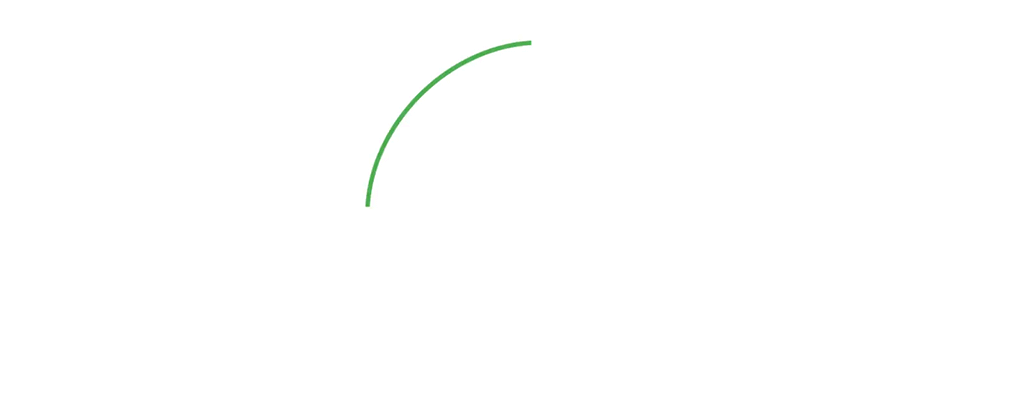
Let’s break it down step by step:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rotating Circle Border</title>
<style>
body
{
margin: 0 auto;
width: 500px;
}
.circle {
margin-top:200px;
width: 400x;
height: 400px;
border: 7px solid transparent;
border-radius: 50%;
border-top-color: #4caf50;
animation: rotate 2s linear infinite;
}
@keyframes rotate {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div class="circle"></div>
</body>
</html>
border defines the border of the circle. It is initially set to a fixed transparency of 5 pixels. This means that the border is initially invisible (transparent) but has a width of 5 pixels.
the border radius is set to 50%, which rounds the border to form a circle.
the top color of the border is set from the top edge of the circle color. In this case it is set to #4caf50 which is shaded green.
The animation property is used to rotate the animation.
@keyframes rotate defines the rotate animation:
- from specifies the starting state of the animation, where the circle is rotated 0deg (i.e., no rotation).
- to specifies the ending state of the animation, where the circle is rotated 360deg (i.e., one full rotation).
Explanation:
- When you load this HTML file in a web browser, it displays a webpage with a single circular element (div) that has a rotating border.
- The border of the circle rotates continually around its center due to the rotate animation defined in the CSS.
- The animation duration is set to 2 seconds, allowing the circle to complete a full rotation in that time.
- The animation timing function is set to linear, ensuring constant rotation speed during the animation.
- The infinite keyword ensures that the animation repeats indefinitely.
2. Stylish CSS Circular Progress with a Percentage Text Indicator
Output :
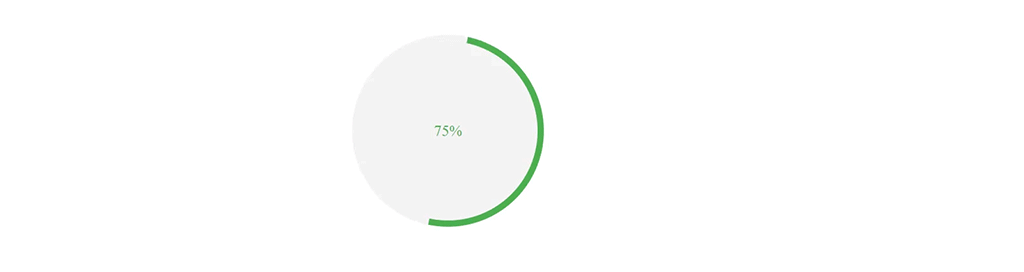
This code generates a css circular progress bar with a gradient overlay that transitions from 0% to 100%. The progress percentage appears in the centre of the bar.
Let’s break it down:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Stylish Circular Progress Bar</title>
<style>
body
{
width:500px;
margin:0 auto;
margin-top: 200px;
}
.progress {
width: 300px;
height: 300px;
border-radius: 50%;
position: relative;
background: #f3f3f3;
overflow: hidden;
}
.progress::before {
content: '';
width: 100%;
height: 100%;
border-radius: 50%;
background: linear-gradient(to right, #4caf50 50%, transparent 50%, transparent);
position: absolute;
top: 0;
left: 0;
animation: animate 5s linear infinite;
}
.inner-circle {
width: calc(100% - 20px);
height: calc(100% - 20px);
border-radius: 50%;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: #f3f3f3;
}
.progress-text {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
font-size: 24px;
color: #4caf50;
}
@keyframes animate {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div class="progress">
<div class="inner-circle"></div>
<div class="progress-text">75%</div>
</div>
</body>
</html>
- .progress::before: Styles the animated gradient progress overlay. It creates a circular overlay with the same dimensions as the .progress container and applies a linear gradient that transitions from green (#4caf50) to transparent. This overlay rotates continuously using CSS animation (@keyframes animate).
- .inner-circle: Styles the inner circle of the progress bar. It creates a slightly smaller circle to act as a background for the progress text. It’s positioned in the center of the .progress container using position: absolute and transform: translate(-50%, -50%).
- .progress-text: Styles the percentage text that appears in the centre of the progress bar. It increases the font size to 24px, changes the colour to green (#4caf50), and puts it in the centre of the page.Progress container with position: absolute and transform: translate(-50%, -50%).
- @keyframes animate : defines a CSS animation. It rotates the progress overlay from 0 to 360 degrees, giving the illusion of progress.
3. Stylish CSS Circular Progress Bar with a Red-to-Black Gradient that Fills a Circular Area Over Time
This code creates a visually appealing progress indicator with a dynamic red-to-black gradient filling a circular area, accompanied by a text indicating the progress percentage.
Output :
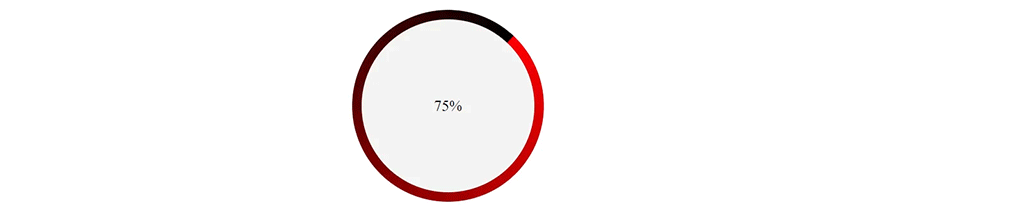
Let’s break it down:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Progress Indicator with Red to Black Gradient</title>
<style>
body
{
width: 500px;
margin: 0 auto;
margin-top: 200px;
}
.progress {
width: 300px;
height: 300px;
border-radius: 50%;
position: relative;
background: #f3f3f3;
overflow: hidden;
}
.progress::before {
content: '';
width: 100%;
height: 100%;
border-radius: 50%;
background: conic-gradient(from -90deg, red, black);
position: absolute;
top: 0;
left: 0;
animation: animate 5s linear infinite;
}
.inner-circle {
width: calc(100% - 30px);
height: calc(100% - 30px);
border-radius: 50%;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: #f3f3f3;
}
.progress-text {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
font-size: 24px;
color: #0c0c0c; /* Text color */
}
@keyframes animate {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div class="progress">
<div class="inner-circle"></div>
<div class="progress-text">75%</div>
</div>
</body>
</html>
- Circular Progress Indicator: The progress indicator is designed as a circular element using the .progress class with a specified width and height.
- Red to Black Gradient Animation: The main attraction of this progress indicator is the red to black gradient animation achieved through the use of conic-gradient in the .progress::before pseudo-element. This creates a visually appealing effect resembling a loading bar.
- Text Display: The percentage completion is displayed at the center of the progress indicator using the .progress-text class.
- Animation: The animation is defined using keyframes (@keyframes animate) to rotate the gradient from 0 to 360 degrees, creating a seamless looping effect.
4.Stylish CSS Circular Progress Bar with a Circle Using CSS animations and Gradients
code results in a CSS circular progress bar with a gradient background that smoothly transitions from a lighter to a darker shade of red, and it continuously rotates in a clockwise direction, giving the appearance of an animated progress indicator.
Output :
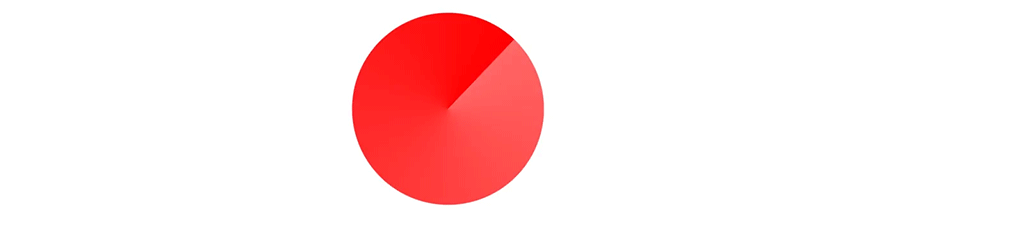
Let’s break it down:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Animated Progress Bar with Red Circle</title>
<style>
body
{
width: 500px;
margin: 0 auto;
margin-top: 200px;
}
.progress {
width: 300px;
height: 300px;
border-radius: 50%;
background: conic-gradient(from 0deg at 50% 50%, #ff6666, #ff0000);
animation: rotate 3s linear infinite;
}
@keyframes rotate {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
</style>
</head>
<body>
<div class="progress"></div>
</body>
</html>
- .progress: This CSS class styles the progress bar container. It sets the width and height to 200 pixels, giving it a circular shape by setting the border-radius property to 50%. The background is created using a conic-gradient, which produces a gradient effect starting from one point and radiating outward in a circular manner. In this case, the gradient starts from the center (50% 50%) and progresses from light red (#ff6666) to dark red (#ff0000).
- @keyframes rotate: This CSS animation keyframe defines the behavior of the rotation animation. It specifies that the element should rotate from 0 degrees to 360 degrees, creating a full circle. The animation lasts for 3 seconds and repeats infinitely (infinite).
Best Practices for Implementation in CSS progress bars
While CSS progress bars provide extensive flexibility and customisation options, it is essential to follow recommended practices for maximum efficiency and accessibility:
1. Semantics Matter:
Use semantic HTML elements to ensure compatibility with assistive technologies and improve accessibility for users with disabilities.
2. Responsive Design:
Design progress bars with responsiveness in mind, ensuring that they adapt smoothly to different screen sizes and devices.
3. Performance Optimization:
Reduce unnecessary styling and animations to improve efficiency, especially on mobile devices with limited resources.
4.Accessibility Considerations:
Make progress bars accessible to all users by including alternative text or aria attributes for screen readers.
Conclusion
CSS progress bars are useful tools that help developers design visually appealing and functioning user experiences. Developers can customise the appearance and behaviour of progress bars to their design preferences, improving the overall user experience. By following best practices for implementation, developers may guarantee that progress bars are accessible, performant, and smoothly incorporated into their web apps. With this book, you’ll be well-prepared to grasp CSS progress bars and take your web development projects to new heights.