Table of Contents
Understanding CSS Variables
Understanding CSS variables requires looking into the concept of cascading style sheets (CSS) and how variables provide a more efficient and flexible method of creating web pages. CSS variables, often known as custom properties, enable developers to build reusable CSS values that may be applied throughout a stylesheet. This not only improves consistency, but also allows for easy maintenance and upgrades across a website or application.
CSS is a language that describes the display of a document written in a markup language such as HTML. It allows you to alter the layout, colors, fonts, and other visual elements of web pages. Traditionally, CSS values are hard-coded into the stylesheet. For example, if a developer wishes to use a given color numerous times in a stylesheet, they must manually update each instance if the color changes. This can be time-consuming and error-prone, particularly with larger projects.
By introducing the idea of variables—a concept that developers from other programming languages are likely familiar with—CSS variables solve this problem. They let programmers declare values only once and utilize them all throughout the stylesheet. This increases the code’s readability and organization while also making it easier to maintain.
Declaration and Syntax of CSS Variables
In the CSS rules, we declare variables for the main part of the document, which is often called the :root element. This allows the variable to be used everywhere in the document. However, you can also choose to only focus on certain parts of the document by specifying it in a different selector.
Learn more about how to declare CSS variables – CSS Variables : The Key to Empowering Your Stylesheets #1.
:root {
--primary-color:#ff0000;
}
Using CSS Variables
CSS variables Once defined, can be applied anywhere in the style sheet using the var() function. For example:
.header {
color: var(--primary-color);
}
In this example, the text color of elements with the .header class is set to the value stored in the –primary-color variable..
Unveiling the HTML Skeleton
Output of example :
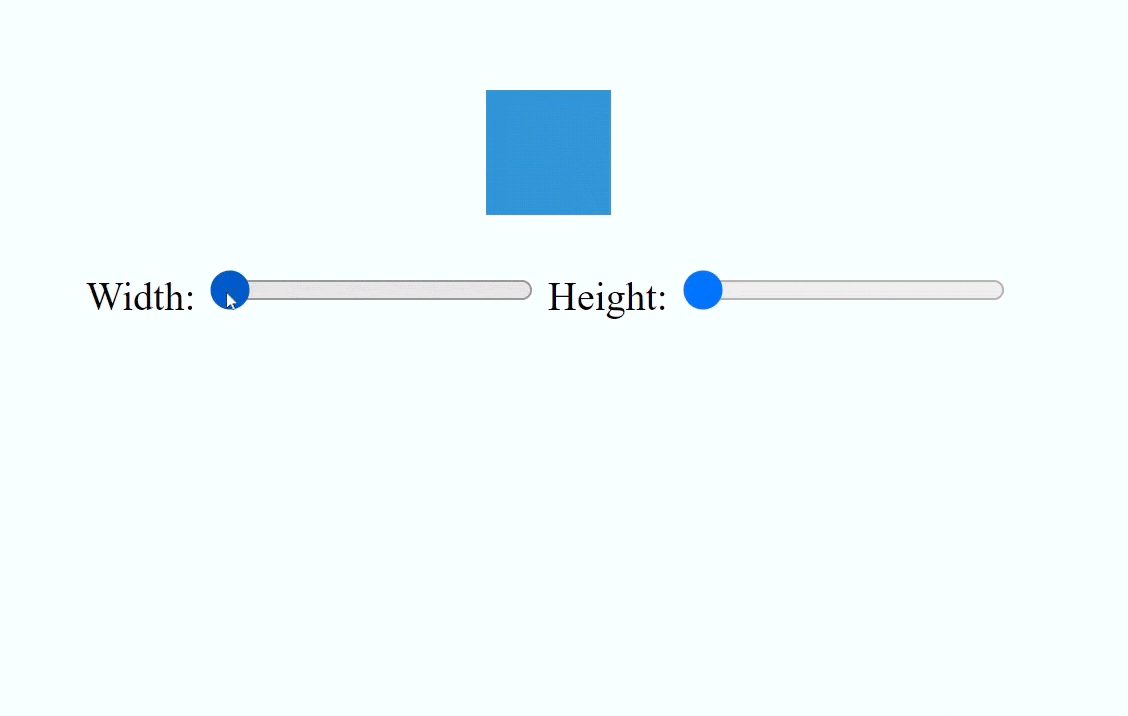
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Meta tags and title -->
<title>CSS Variables Example 2</title>
<style>
/* add CSS Code Here */
</style>
</head>
<body>
<!-- Container div -->
<div class="container">
<!-- Box element -->
<div class="box"></div>
<!-- Input range for width -->
<label for="widthRange">Width:</label>
<input type="range" id="widthRange" min="50" max="300" value="150">
<!-- Input range for height -->
<label for="heightRange">Height:</label>
<input type="range" id="heightRange" min="50" max="300" value="150">
</div>
<!-- JavaScript code -->
<script>
//Add JavaScript code here
</script>
</body>
</html>
Make use of the power of CSS variables
In the code, we define two CSS variables –box-width and –box-height using the :root selector. These variables act as placeholders to hold values for the size of the box element
:root {
--box-width: 150px;
--box-height: 150px;
}
.box {
width: var(--box-width);
height: var(--box-height);
background-color: #3498db;
margin: 20px auto;
transition: width 0.5s, height 0.5s; /* Smooth transition effect */
}
- The :root pseudo-class selection follows the HTML document’s root element. It is used here to specify global CSS variables that can be accessed from anywhere in the document.
- –box-width and –box-height are CSS variables that specify the width and height of the box.
- width: var(–box-width); and height: var(–box-height); respectively set the width of elements with the class box to the values contained in the –box-width and –box-height variables.
- Transition: width 0.5s, height 0.5s; – This line creates a smooth transition effect for changing the width and height characteristics of elements with the class box. Both characteristics have a transition duration of 0.5 seconds.
Dynamic Interaction of CSS Variable with JavaScript
JavaScript is placed between the tags <script>…</script>. The event listener is added to the width and height range sliders and waits for user input. When the user edits the slider, the corresponding CSS variable is updated using document.documentElement.style.setProperty()..
document.getElementById('widthRange').addEventListener('input', function() {
var widthValue = this.value + 'px';
document.documentElement.style.setProperty('--box-width', widthValue);
});
document.getElementById('heightRange').addEventListener('input', function() {
var heightValue = this.value + 'px';
document.documentElement.style.setProperty('--box-height', heightValue);
});
Event Listener for width Range Input
- document.getElementById(‘widthRange’):This code selects the HTML element with the ID ‘widthRange’. This is usually a <input> element of type range.
- Add an event listener (‘input’, function() {… }): This line adds a listener to the selected element’s input event. This event is generated whenever the input value changes.
- function() { … }: This is the event handler function that is called whenever the input event occurs.
Event Listener for Height Range Input
This function works in the same way as the above. The only change is to change the height of the box.
- document.getElementById(‘heightRange’): This code selects the HTML element with the ID ‘heightRange’. This is usually a <input> element of type range.
- addEventListener(‘input’, function() { … }): This line adds a listener to the selected element’s input event. This event is generated whenever the input value changes.
- var widthValue = this.value + ‘px’;: This line retrieves the input element’s current value (widthRange) and concatenates it with the string ‘px’ to generate a valid CSS width value.
- document.documentElement.style.setProperty(‘–box-width’, widthValue);: This line sets the CSS variable –box-width to the previously determined widthValue. The width of the box is updated in real time as the user adjusts the range input.
In the event handler function:
- var heightValue = this.value + ‘px’;: This line gets the current value of the heightRange input and concatenates it with the string ‘px’ to create a valid CSS height value.
- document.documentElement.style.setProperty(‘–box-height’, heightValue);: This line sets the value of the –box-height CSS variable to heightValue, which updates the height of the box (as specified in the CSS variable) in real-time as the user adjusts the range input.
As the user interacts with the width and height sliders, the .box element automatically resizes in real time using CSS variables and JavaScript. The simple interaction between user input and dynamic styles shows the strength and power of CSS variables in modern web development.
Conclusion:
Finally, CSS variables offer a new approach to web application development, enabling developers to easily create flexible and adaptive user interfaces. Developers can use CSS variables’ dynamic capabilities to create engaging experiences that respond to user preferences and activities. So, why wait? Step into the world of CSS variables and discover limitless possibilities for your website projects!